Basic Operators
An operator is a special symbol or phrase that you use to check, change, or combine values. For example, the addition operator
(+
) adds two numbers together (as in let i = 1 + 2
). More complex examples include the logical AND
operator &&
(as in if enteredDoorCode && passedRetinaScan)
and the compound assignment
operator +=
, which is a shortcut to increase the value of i
.
Flax supports most standard C operators and improves several capabilities to eliminate common coding errors. The assignment
operator (=
) does not return a value, to prevent it from being mistakenly used when the equal to operator
(==
) is intended. Arithmetic operators (+
, -
, *
, /
,
%
and so forth) can be configured to check for overflow or underflow errors, when performance is not critical.
Additionally, unlike C, Flax lets you perform remainder (%
) calculations on floating-point numbers.
This chapter describes the common operators in Flax. Advanced Operators covers Flax's advanced operators, and describes how to define your own custom operators and implement the standard operators for your own custom types.
Terminology
Operators are unary, binary, or ternary:
-
Unary operators operate on a single target (such as
-a
). Unary prefix operators appear immediately before their target (such as!b
), and unary postfix operators appear immediately after their target (such asi++
). -
Binary operators operate on two targets (such as
2 + 3
) and are infix because they appear in between their two targets. -
Ternary operators operate on three targets. Like C, Flax has only one ternary operator, the ternary conditional
operator (
a ? b : c
).
The values that operators affect are operands. In the expression 1 + 2
, the +
symbol is a binary
operator and its two operands are the values 1
and 2
.
Assignment Operator
The assignment operator (a = b
) initializes or updates the value of a
with the value of b
:
let b = 10
var a = 5
a = b
// a is now equal to 10
If the right side of the assignment is a tuple with multiple values, its elements can be decomposed into multiple constants or variables at once:
let (x, y) = (1, 2)
// x is equal to 1, and y is equal to 2
This is known as tuple decomposition, and is a highly powerful tool.
Unlike the assignment operator in languages such as C and Java, the assignment operator in Flax does not return a value. Hence, the following statements are invaild:
if x = y {
// this is not valid, because x = y does not return a value
}
var b = 30
var c = 10
var k = b = c // this is not valid.
This feature prevents the assignment operator (=
) from being used by accident when the equal to operator
(==
) is actually intended.
Arithmetic Operators
Flax supports the four basic arithmetic operators on all builtin numeric types:
- (
+
) Addition - (
-
) Subtraction - (
*
) Multiplication - (
/
) Division
Additionally, the unicode characters ×
and ÷
can be used in place of multiplcation (*
)
and division (/
) respectively.
The addition operator is also supported for String concatenation:
let str = "Hello, " + "World!"
printf("%s\n", str)
// prints "Hello, World!"
Remainder Operator
The remainder operator (a % b
) works out how many multiples of b
will fit inside a
and
returns the value that is left over (known as the remainder).
Here’s how the remainder operator works. To calculate 9 % 4
, you first work out how many 4
s will fit
inside 9
:

You can fit two 4
s inside 9
, and the remainder is 1
(shown in orange).
This would be written in Flax as:
let m = 9 % 4 // equals 1
To determine the answer for a % b
, the %
operator calculates the following equation and returns
remainder
as its output:
a = (b x some multiplier) + remainder
where some multiplier
is the largest number of multiples of b
that will fit inside a
.
Inserting 9
and 4
into this equation yields:
9 = (4 x 2) + 1
The same method is applied when calculating the remainder for a negative value of a
:
let m = -9 % 4 // equals -1
Inserting -9
and 4
into the equation yields:
-9 = (4 x -2) + -1
giving a remainder value of -1
.
The sign of b
is ignored for negative values of b
. This means that a % b
and
a % -b
always give the same answer.
Floating-Point Remainder Operator
Unlike the remainder operator in C, Flax's remainder operator can also operate on floating-point numbers:
let m = 8 % 2.5 // equals 0.5
In this example, 8
divided by 2.5
equals 3
, with a remainder of 0.5
, so
the remainder operator returns a Double
value of 0.5
.
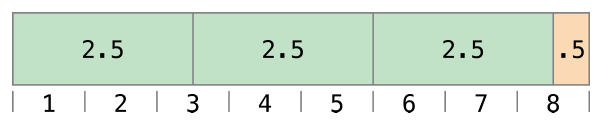
Unary Minus Operator
The sign of a numeric value can be toggled using a prefixed -
, known as the unary minus operator:
let three = 3
let minusThree = -three // minusThree equals -3
let plusThree = -minusThree // plusThree equals 3, or "minus minus three"
The unary minus operator (-
) is prepended directly before the value it operates on, without any white space.
Unary Plus Operator
The unary plus operator (+
) simply returns the value it operates on, without any change:
let minusSix = -6
let alsoMinusSix = +minusSix // alsoMinusSix equals -6
Although the unary plus operator doesn’t actually do anything, you can use it to provide symmetry in your code for positive numbers when also using the unary minus operator for negative numbers.
Compound Assignment Operators
Like C, Flax provides compound assignment operators that combine assignment (=
) with another operation.
One example is the addition assignment operator (+=
):
var a = 1
a += 2
// a is now equal to 3
The expression a += 2
is shorthand for a = a + 2
. Effectively, the addition and the assignment are
combined into one operator that performs both tasks at the same time.
Here is a list of the supported compound assignment operators:
- (
+=
) Plus equals - (
-=
) Minus equals - (
*=
) Times equals - (
/=
) Divide equals - (
%=
) Mod equals - (
&=
) Binary And equals - (
|=
) Binary Or equals - (
^=
) Binary Xor equals - (
<<=
) Shift left equals - (
>>=
) Shift right equals
Increment and Decrement Operators
Like C, Flax provides an increment operator (++
) and a decrement operator (--
) as a shortcut to increase
or decrease the value of a numeric variable by 1
. You can use these operators with variables of any integer or
floating-point type.
var i = 0
++i // i now equals 1
Each time you call ++i
, the value of i
is increased by 1
. Essentially, ++i
is
shorthand for saying i += 1
. Likewise, --i
can be used as shorthand for i -= 1
.
The ++
and --
symbols can be used as prefix operators or as postfix operators. ++i
and
i++
are both valid ways to increase the value of i
by 1
. Similarly, --i
and
i--
are both valid ways to decrease the value of i
by 1
.
Naturally, these operators modify i
and also return a value. If you only want to increment or decrement the value stored
in i
, you can ignore the returned value. However, if you do use the returned value, it will be different based
on whether you used the prefix or postfix version of the operator, according to the following rules:
- If the operator is written before the variable, it modifies the variable before returning its value.
- If the operator is written after the variable, it modifies the variable after returning its value.
For example:
var a = 0
let b = ++a
// a and b are now both equal to 1
let c = a++
// a is now equal to 2, but c has been set to the pre-increment value of 1
In the example above, let b = ++a
increments a before returning its value. This is why both a and b are equal to the
new value of 1
.
However, let c = a++
increments a after returning its value. This means that c
gets the old value of
1
, and a is then updated to equal 2
.
Unless you need the specific behavior of i++
, it is recommended that you use ++i
and --i
in all cases, because they have the typical expected behavior of modifying i and returning the result.
Comparison Operators
Flax supports all of the standard C comparison operators:
- (
a < b
) Less than - (
a > b
) Greater than - (
a == b
) Equal to - (
a != b
) Not equal to - (
a <= b
) Less than or equal to - (
a >= b
) Greater than or equal to
Each of the comparison operators returns a Bool
value to indicate whether or not the statement is true:
1 < 2 // true, because 1 is less than 2
2 > 1 // true, because 2 is greater than 1
1 == 1 // true, because 1 is equal to 1
2 != 1 // true, because 2 is not equal to 1
2 <= 1 // false, because 2 is not less than or equal to 1
1 >= 1 // true, because 1 is greater than or equal to 1
Comparison operators are often used in conditional statements, such as the if
statement:
let name = "world"
if name == "world" {
print("hello, world")
} else {
print("I'm sorry \(name), but I don't recognize you")
}
// prints "hello, world", because name is indeed equal to "world"
For more on the if
statement, see Control Flow.
Ternary Conditional Operator
The ternary conditional operator is a special operator with three parts, which takes the form
question ? answer1 : answer2
. It is a shortcut for evaluating one of two expressions based on whether question is
true
or false
. If question is true
, it evaluates answer1
and returns its value;
otherwise, it evaluates answer2
and returns its value.
The ternary conditional operator is shorthand for the code below:
if question
{
answer1
}
else
{
answer2
}
Here’s an example, which calculates the height for a table row. The row height should be 50 points taller than the content height if the row has a header, and 20 points taller if the row doesn’t have a header:
let contentHeight = 40
let hasHeader = true
let rowHeight = contentHeight + (hasHeader ? 50 : 20)
// rowHeight is equal to 90
The preceding example is shorthand for the code below:
let contentHeight = 40
let hasHeader = true
var rowHeight = contentHeight
if hasHeader
{
rowHeight = rowHeight + 50
}
else
{
rowHeight = rowHeight + 20
}
// rowHeight is equal to 90
The first example’s use of the ternary conditional operator means that rowHeight
can be set to the correct value on a
single line of code. This is more concise than the second example, and removes the need for rowHeight
to be a variable,
because its value does not need to be modified within an if statement.
The ternary conditional operator provides an efficient shorthand for deciding which of two expressions to consider. Use the ternary conditional operator with care, however. Its conciseness can lead to hard-to-read code if overused. Avoid combining multiple instances of the ternary conditional operator into one compound statement.
Logical Operators
Logical operators modify or combine the Boolean logic values true and false. Flax supports the three standard logical operators found in C-based languages:
- (
!a
) Logical NOT - (
a || b
) Logical AND - (
a && b
) Logical OR
Logical NOT Operator
The logical NOT operator (!a
) inverts a Boolean value so that true
becomes false
,
and false
becomes true
.
The logical NOT operator is a prefix operator, and appears immediately before the value it operates on, without any white space. It can be read as "not a", as seen in the following example:
let allowedEntry = false
if !allowedEntry
{
print("ACCESS DENIED")
}
// prints "ACCESS DENIED"
The phrase if !allowedEntry
can be read as "if not allowed entry". The subsequent line is only executed if "not allowed
entry" is true
; that is, if allowedEntry
is false
.
As in this example, careful choice of Boolean constant and variable names can help to keep code readable and concise, while avoiding double negatives or confusing logic statements.
Logical AND Operator
The logical AND operator (a && b
) creates logical expressions where both values must be true
for the overall expression to also be true
.
If either value is false
, the overall expression will also be false
. In fact, if the first value is
false
, the second value won’t even be evaluated, because it can’t possibly make the overall expression equate to
true
. This is known as short-circuit evaluation.
This example considers two Bool
values and only allows access if both values are true
:
let enteredDoorCode = true
let passedRetinaScan = false
if enteredDoorCode && passedRetinaScan
{
print("Welcome!")
}
else
{
print("ACCESS DENIED")
}
// prints "ACCESS DENIED"
Logical OR Operator
The logical OR operator (a || b
) is an infix operator made from two adjacent pipe characters. You use it to create
logical expressions in which only one of the two values has to be true
for the overall expression to be
true
.
Like the Logical AND operator above, the Logical OR operator uses short-circuit evaluation to consider its expressions. If the left
side of a Logical OR expression is true
, the right side is not evaluated, because it cannot change the outcome of the
overall expression.
In the example below, the first Bool
value (hasDoorKey
) is false
, but the second value
(knowsOverridePassword
) is true. Because one value is true
, the overall expression also evaluates to
true
, and access is allowed:
let hasDoorKey = false
let knowsOverridePassword = true
if hasDoorKey || knowsOverridePassword
{
print("Welcome!")
}
else
{
print("ACCESS DENIED")
}
// prints "Welcome!"
Combining Logical Operators
You can combine multiple logical operators to create longer compound expressions:
if enteredDoorCode && passedRetinaScan || hasDoorKey || knowsOverridePassword
{
print("Welcome!")
}
else
{
print("ACCESS DENIED")
}
// prints "Welcome!"
This example uses multiple &&
and ||
operators to create a longer compound expression. However,
the &&
and ||
operators still operate on only two values, so this is actually three smaller
expressions chained together. The example can be read as:
If we’ve entered the correct door code and passed the retina scan, or if we have a valid door key, or if we know the emergency override password, then allow access.
Based on the values of enteredDoorCode
, passedRetinaScan
, and hasDoorKey
, the first two
subexpressions are false
. However, the emergency override password is known, so the overall compound expression still
evaluates to true
.
Explicit Parentheses
It is sometimes useful to include parentheses when they are not strictly needed, to make the intention of a complex expression easier to read. In the door access example above, it is useful to add parentheses around the first part of the compound expression to make its intent explicit:
if (enteredDoorCode && passedRetinaScan) || hasDoorKey || knowsOverridePassword {
print("Welcome!")
}
else
{
print("ACCESS DENIED")
}
// prints "Welcome!"
The parentheses make it clear that the first two values are considered as part of a separate possible state in the overall logic. The output of the compound expression doesn’t change, but the overall intention is clearer to the reader. Readability is always preferred over brevity; use parentheses where they help to make your intentions clear.